Semaphor
Definition
Mutex is just an object while Semaphore is an integer. Mutex has no subtype whereas Semaphore has two types, which are counting semaphore and binary semaphore. Semaphore supports wait and signal operations modification, whereas Mutex is only modified. SEMAPHOR works best in the newest and last prior version of these browsers: Google Chrome. To get the best SEMAPHOR experience and security updates, upgrade to the latest version of Chrome. Firefox; Internet Explorer and Microsoft Edge. Le Havre gateway to the Oceans Le Havre is the first major port commercial ships encounter coming up the English Channel. The capitainerie is an imposing tower built to control the movement of ships around the port. It is built close to the site of the port’s original semaphore which was destroyed during.
Limits the number of threads that can access a resource or pool of resources concurrently.
- Attributes
Examples
The following code example creates a semaphore with a maximum count of three and an initial count of zero. The example starts five threads, which block waiting for the semaphore. The main thread uses the Release(Int32) method overload to increase the semaphore count to its maximum, allowing three threads to enter the semaphore. Each thread uses the Thread.Sleep method to wait for one second, to simulate work, and then calls the Release() method overload to release the semaphore. Each time the semaphore is released, the previous semaphore count is displayed. Console messages track semaphore use. The simulated work interval is increased slightly for each thread, to make the output easier to read.
Remarks
Use the Semaphore class to control access to a pool of resources. Threads enter the semaphore by calling the WaitOne method, which is inherited from the WaitHandle class, and release the semaphore by calling the Release method.
The count on a semaphore is decremented each time a thread enters the semaphore, and incremented when a thread releases the semaphore. When the count is zero, subsequent requests block until other threads release the semaphore. When all threads have released the semaphore, the count is at the maximum value specified when the semaphore was created.
There is no guaranteed order, such as FIFO or LIFO, in which blocked threads enter the semaphore.
A thread can enter the semaphore multiple times, by calling the WaitOne method repeatedly. To release some or all of these entries, the thread can call the parameterless Release() method overload multiple times, or it can call the Release(Int32) method overload that specifies the number of entries to be released.
The Semaphore class does not enforce thread identity on calls to WaitOne or Release. It is the programmer's responsibility to ensure that threads do not release the semaphore too many times. For example, suppose a semaphore has a maximum count of two, and that thread A and thread B both enter the semaphore. If a programming error in thread B causes it to call Release twice, both calls succeed. The count on the semaphore is full, and when thread A eventually calls Release, a SemaphoreFullException is thrown.
Semaphores are of two types: local semaphores and named system semaphores. If you create a Semaphore object using a constructor that accepts a name, it is associated with an operating-system semaphore of that name. Named system semaphores are visible throughout the operating system, and can be used to synchronize the activities of processes. You can create multiple Semaphore objects that represent the same named system semaphore, and you can use the OpenExisting method to open an existing named system semaphore.
A local semaphore exists only within your process. It can be used by any thread in your process that has a reference to the local Semaphore object. Each Semaphore object is a separate local semaphore.
Constructors
Semaphore(Int32, Int32) | Initializes a new instance of the Semaphore class, specifying the initial number of entries and the maximum number of concurrent entries. |
Semaphore(Int32, Int32, String) | Initializes a new instance of the Semaphore class, specifying the initial number of entries and the maximum number of concurrent entries, and optionally specifying the name of a system semaphore object. |
Semaphore(Int32, Int32, String, Boolean) | Initializes a new instance of the Semaphore class, specifying the initial number of entries and the maximum number of concurrent entries, optionally specifying the name of a system semaphore object, and specifying a variable that receives a value indicating whether a new system semaphore was created. |
Semaphore(Int32, Int32, String, Boolean, SemaphoreSecurity) | Initializes a new instance of the Semaphore class, specifying the initial number of entries and the maximum number of concurrent entries, optionally specifying the name of a system semaphore object, specifying a variable that receives a value indicating whether a new system semaphore was created, and specifying security access control for the system semaphore. |
Fields
WaitTimeout | Indicates that a WaitAny(WaitHandle[], Int32, Boolean) operation timed out before any of the wait handles were signaled. This field is constant. (Inherited from WaitHandle) |
Properties
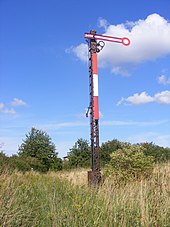
Handle | Gets or sets the native operating system handle. (Inherited from WaitHandle) |
SafeWaitHandle | Gets or sets the native operating system handle. (Inherited from WaitHandle) |
Methods
Close() | Releases all resources held by the current WaitHandle. (Inherited from WaitHandle) |
CreateObjRef(Type) | Creates an object that contains all the relevant information required to generate a proxy used to communicate with a remote object. (Inherited from MarshalByRefObject) |
Dispose() | Releases all resources used by the current instance of the WaitHandle class. (Inherited from WaitHandle) |
Dispose(Boolean) | When overridden in a derived class, releases the unmanaged resources used by the WaitHandle, and optionally releases the managed resources. (Inherited from WaitHandle) |
Equals(Object) | Determines whether the specified object is equal to the current object. (Inherited from Object) |
GetAccessControl() | Gets the access control security for a named system semaphore. |
GetHashCode() | Serves as the default hash function. (Inherited from Object) |
GetLifetimeService() | Obsolete. Retrieves the current lifetime service object that controls the lifetime policy for this instance. (Inherited from MarshalByRefObject) |
GetType() | Gets the Type of the current instance. (Inherited from Object) |
InitializeLifetimeService() | Obtains a lifetime service object to control the lifetime policy for this instance. (Inherited from MarshalByRefObject) |
MemberwiseClone() | Creates a shallow copy of the current Object. (Inherited from Object) |
MemberwiseClone(Boolean) | Creates a shallow copy of the current MarshalByRefObject object. (Inherited from MarshalByRefObject) |
OpenExisting(String) | Opens the specified named semaphore, if it already exists. |
OpenExisting(String, SemaphoreRights) | Opens the specified named semaphore, if it already exists, with the desired security access. |
Release() | Exits the semaphore and returns the previous count. |
Release(Int32) | Exits the semaphore a specified number of times and returns the previous count. |
SetAccessControl(SemaphoreSecurity) | Sets the access control security for a named system semaphore. |
ToString() | Returns a string that represents the current object. (Inherited from Object) |
TryOpenExisting(String, Semaphore) | Opens the specified named semaphore, if it already exists, and returns a value that indicates whether the operation succeeded. |
TryOpenExisting(String, SemaphoreRights, Semaphore) | Opens the specified named semaphore, if it already exists, with the desired security access, and returns a value that indicates whether the operation succeeded. |
WaitOne() | Blocks the current thread until the current WaitHandle receives a signal. (Inherited from WaitHandle) |
WaitOne(Int32) | Blocks the current thread until the current WaitHandle receives a signal, using a 32-bit signed integer to specify the time interval in milliseconds. (Inherited from WaitHandle) |
WaitOne(Int32, Boolean) | Blocks the current thread until the current WaitHandle receives a signal, using a 32-bit signed integer to specify the time interval and specifying whether to exit the synchronization domain before the wait. (Inherited from WaitHandle) |
WaitOne(TimeSpan) | Blocks the current thread until the current instance receives a signal, using a TimeSpan to specify the time interval. (Inherited from WaitHandle) |
WaitOne(TimeSpan, Boolean) | Blocks the current thread until the current instance receives a signal, using a TimeSpan to specify the time interval and specifying whether to exit the synchronization domain before the wait. (Inherited from WaitHandle) |
Explicit Interface Implementations
IDisposable.Dispose() | This API supports the product infrastructure and is not intended to be used directly from your code. Releases all resources used by the WaitHandle. (Inherited from WaitHandle) |
Extension Methods
GetAccessControl(Semaphore) | Returns the security descriptors for the specified |
SetAccessControl(Semaphore, SemaphoreSecurity) | Sets the security descriptors for the specified semaphore. |
GetSafeWaitHandle(WaitHandle) | Gets the safe handle for a native operating system wait handle. |
SetSafeWaitHandle(WaitHandle, SafeWaitHandle) | Sets a safe handle for a native operating system wait handle. |
Applies to
Thread Safety
This type is thread safe.

Also found in: Thesaurus, Encyclopedia.
sem·a·phore
(sĕm′ə-fôr′)n.semaphore
Cached
(ˈsɛməˌfɔː) nsem•a•phore
(ˈsɛm əˌfɔr, -ˌfoʊr)n., v. -phored, -phor•ing.n.
semaphore
semaphore
Past participle: semaphored
Semaphore Timeout Period Has Expired

Imperative |
---|
semaphore |
semaphore |
Present |
---|
I semaphore |
you semaphore |
he/she/it semaphores |
we semaphore |
you semaphore |
they semaphore |
Preterite |
---|
I semaphored |
you semaphored |
he/she/it semaphored |
we semaphored |
you semaphored |
they semaphored |
Present Continuous |
---|
I am semaphoring |
you are semaphoring |
he/she/it is semaphoring |
we are semaphoring |
you are semaphoring |
they are semaphoring |

Present Perfect |
---|
I have semaphored |
you have semaphored |
he/she/it has semaphored |
we have semaphored |
you have semaphored |
they have semaphored |
Past Continuous |
---|
I was semaphoring |
you were semaphoring |
he/she/it was semaphoring |
we were semaphoring |
you were semaphoring |
they were semaphoring |
Past Perfect |
---|
I had semaphored |
you had semaphored |
he/she/it had semaphored |
we had semaphored |
you had semaphored |
they had semaphored |
Future |
---|
I will semaphore |
you will semaphore |
he/she/it will semaphore |
we will semaphore |
you will semaphore |
they will semaphore |
Future Perfect |
---|
I will have semaphored |
you will have semaphored |
he/she/it will have semaphored |
we will have semaphored |
you will have semaphored |
they will have semaphored |
Future Continuous |
---|
I will be semaphoring |
you will be semaphoring |
he/she/it will be semaphoring |
we will be semaphoring |
you will be semaphoring |
they will be semaphoring |
Present Perfect Continuous |
---|
I have been semaphoring |
you have been semaphoring |
he/she/it has been semaphoring |
we have been semaphoring |
you have been semaphoring |
they have been semaphoring |
Future Perfect Continuous |
---|
I will have been semaphoring |
you will have been semaphoring |
he/she/it will have been semaphoring |
we will have been semaphoring |
you will have been semaphoring |
they will have been semaphoring |
Past Perfect Continuous |
---|
I had been semaphoring |
you had been semaphoring |
he/she/it had been semaphoring |
we had been semaphoring |
you had been semaphoring |
they had been semaphoring |
Conditional |
---|
I would semaphore |
you would semaphore |
he/she/it would semaphore |
we would semaphore |
you would semaphore |
they would semaphore |
Past Conditional |
---|
I would have semaphored |
you would have semaphored |
he/she/it would have semaphored |
we would have semaphored |
you would have semaphored |
they would have semaphored |
semaphore
Noun | 1. | semaphore - an apparatus for visual signaling with lights or mechanically moving arms apparatus, setup - equipment designed to serve a specific function |
Verb | 1. | semaphore - send signals by or as if by semaphore signal, signalise, signalize, sign - communicate silently and non-verbally by signals or signs; 'He signed his disapproval with a dismissive hand gesture'; 'The diner signaled the waiters to bring the menu' |
2. | semaphore - convey by semaphore, of information communicate, intercommunicate - transmit thoughts or feelings; 'He communicated his anxieties to the psychiatrist' |
semaphore
verbsemaphore
[ˈseməfɔːʳ]semaphore
[ˈsɛməfɔːr]nsemaphore
nsemaphore
[ˈsɛməˌfɔːʳ]nsemaphore
(ˈseməfoː) noun
Link to this page:
